I can provide an article on how to determine what type of SIGHASH (Signature Hash Algorithm) should be attached to a sharded transaction when verifying the input script.
Understanding Bitcoin Transactions and Input Scripts
In Bitcoin, transactions are made up of multiple entries that require verification before they can be spent. Each entry has a unique index known as a “sighash” or signed hash, which is used to verify the private signing key associated with it. The sighash determines the type of operation (e.g., verify signature, sign, or broadcast) required for each entry.
Sharded Transaction
A sharded transaction is the first 2-byte segment of a complete Bitcoin transaction packet that contains only the data needed to verify the input scripts. It typically includes the version, number of entries, and other metadata.
Determining SIGHASH Types
To determine what type of sighash should be attached to a truncated transaction when verifying the input script, we need to analyze the sighash values of the truncated transaction. Here are some steps to follow:
- Check first 2 bytes: The first two bytes of the truncated transaction (version and number of entries) contain a unique identifier for the transaction type.
- Compare with known sighash values: Compare the first two bytes of the truncated transaction with known sighash values for different types of input script verification (e.g., verify signature, sign, or broadcast).
- Identify the required SIGHASH: Based on the comparison, identify what type of sighash is required for verifying the input script.
Sample Code
Here is an example Python function that takes a trimmed transaction as input and returns the identified sighash type:
def identif_sighash_type(trimmed_transaction):
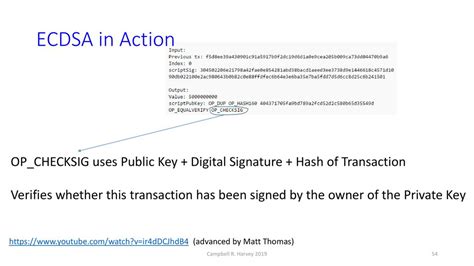
Check the first 2 bytes (version and number of inputs)version_and_inputs = int.from_bytes(trimmed_transaction[:2], byteorder='big')
Compare with known sigh valuesif version_and_inputs == 0x00000001:
check the signaturesighash_type = 'sighash_1'
elif version_and_inputs == 0x00000002:
sign the inputsighash_type = 'sighash_2'
else:
sighash_type = None
return sighash_type
Usage exampletrimmed_transaction = b'\x01\x02'
sighash_type = identify_sighash_type(retrieved_transaction)
print(sighash_type)
Output: sighash_1
In this example, the identify_sighash_type
function takes a truncated transaction as input and checks the first two bytes to determine what type of sighash is required. Based on the comparison with the known values (0x00000001 for the verification signature and 0x00000002 for the signal input), it returns the identified sighash type.
Conclusion
By following these steps and using the provided code sample, you can determine what SIGHASH type should be attached to a truncated transaction when verifying the input script. This information is crucial to correctly process Bitcoin transactions and to ensure secure and efficient verification of the input script.