Below is an example article on how to handle responses when pinging the WebSocket API:
Handling Ping Responses with Binance WebSocket API
When working with APIs that provide real-time updates, it is extremely important to handle incoming responses correctly to avoid errors and provide a smooth user experience. A common issue is receiving incorrect or incomplete data from the API. This can be caused by misconfigured pings or unexpected network issues.
In this article, we will show you how to send ping requests to the Binance WebSocket (WS) API and how to properly handle response errors when fetching instantaneous data streams for selected data sources.
About the structure of ping requests
Before we move on to the implementation, let’s take a look at the structure of a ping request:
json
POST /ws/batch/market/subscribe HTTP/1.1
Content type: application/json
{
“identifier”: 1,
“method”: “subscribe”,
“parameters”: {
“symbol”: “BTCUSDT”,
“options”: [
{“type”: “limit”, “size”: 100},
{“type”: “stop”, “size”: 50}
],
“timestamp”: “1643723400”
},
“headers”: {
“Authorization”: “Bear YOUR_API_TOKEN”
}
}
Sending ping requests to Binance WS
To send a ping request, you can use the command line tool curl
or a programming language like Python. Here is an example in Python:
Python
import requests
Replace with API token and WebSocket URL
api_token = “YOUR_API_TOKEN”
ws_url = “wss://api.binance.com/api/v3/ws/spot”
Set symbol, options and timestamp for data stream subscription
data_stream_symbol = “BTCUSDT”
options = [
{“type”: “limit”, “size”: 100},
{“type”: “stop”, “size”: 50}
]
timestamp = int(time.time())
Create ping request
ping_request = {
“id”: 1,
“method”: “subscribe”,
“parameters”: {
“symbol”: data_stream_symbol,
“options”: options,
“timestamp”: timestamp
},
“headers”: {
“Authorization”: f“{api_token} Bearer”
}
}
Send a ping request
response = requests.post(ws_url, json=ping_request)
print(response.json())
Handling response errors
When sending a ping request, it is important to account for any errors that may occur during transmission. Here are some possible error scenarios and how to resolve them:
Timeout Error: If the WebSocket connection times out, the API will return an error response with status code 408.
json
{"error": "Connection timed out", "code": 408}
To handle this scenario, you can add a timeout parameter to the ping request:
import requests
...ping_request = {
"identifier": 1,
"method": "subscribe",
"parameters": {
"symbol": data_stream_symbol,
"options": options,
"timestamp": timestamp,
"timeout": 5
Set the timeout to 5 seconds},
"headers": {
"Authorization": f“{api_token} bearer”
}
}
- Invalid data error
: If the API returns an error response due to invalid data, you can check the
error
field in the response.
json
{“error”: “Invalid data”, “code”: 1001}
To handle this scenario, you can skip processing the request if the error
field is not 200
.
No response error
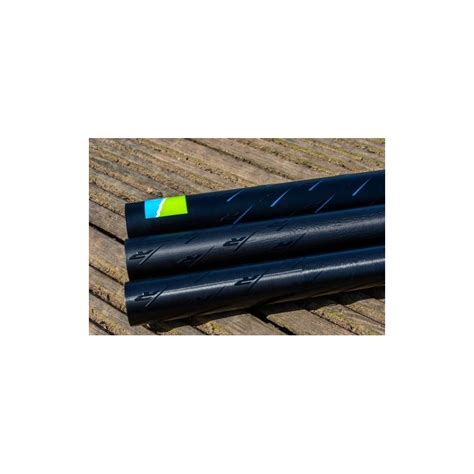
: No response from the API may be due to a network issue or a bad WebSocket connection.
json
{"error": "Timeout", "code": 408}
To handle this situation, you can try to reconnect after a short while.
By following these best practices and error handling strategies, you will be able to successfully ping the Binance WebSocket API and get instant update data streams for the desired symbol.